RecursosGuias
Guias
Voltar | Lista de artigos
Como criar uma Integração com um ERP?
Este documento descreve os passos necessários para criar uma Integração com um ERP com sucesso. Deve ser criada uma Dll com o nome "GlobalSist.WMS.[NomeERP].Integration" que terá de cumprir com uma interface descrita nos seguintes passos.
Pré-Requisitos
- PRIMAVERA Eye Peak;
- Visual Studio 2013 ou superior;
- NET Framework 4.0.
Passo 1 – Criar uma solução
- Depois de abrir o Visual Studio, selecionar File > New > Project.
- Na janela apresentada, expandir na árvore a linguagem pretendida (“C#” ou “VB”).
- Abaixo da linguagem, selecionar Windows Desktop.
- Selecionar o tipo de projeto “Class Library (.NET Framework)".
- Atribuir um nome ao projeto (deve seguir as nomeclatura "GlobalSist.WMS.[NomeERP].Integration"), escolher a localização do projeto e atribuir um nome à solução. Nota: Verificar se a versão da Framework selecionada por baixo do nome da solução é a Framework 4.
Passo 2 – Adicionar Referências
- No Solution Explorer do Visual Studio, selecionar o nó References.
- Clicar com o botão direito do rato e escolher “Add References…”.
- Na janela que é apresentada, clicar no botão “Browse…” e navegar até à pasta de instalação do Eye Peak. Numa instalação por defeito a pasta será: “C:Program Files (x86)PRIMAVERAWMS510Eye Peak WMS”.
- Selecionar os ficheiros: "GlobalSist.Common.dll", "GlobalSist.Logic.dll", "GlobalSist.DataAccess.dll", "GlobalSist.AppServices.dll", "GlobalSist.ServiceProvider.dll", "GlobalSist.Entities.WMS.dll", "GlobalSist.WMS.Integration.dll", "GlobalSist.Server.Jobs.dll" e "GlobalSist.Facades.dll".
- Clicar no botão “Add” e de seguida no botão “OK”.
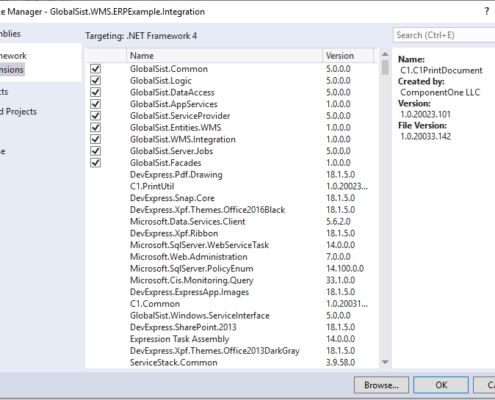
Passo 3 – Criar Class Module "Configuration"
- No nó do projeto carregar com o botão direito do rato, e escolher Add > New Item…
- Na janela apresentada, na árvore expandir a linguagem pretendida (“C#” ou “VB”) e abaixo da linguagem selecionar Code.
- Selecionar o tipo “Class”.
- Indicar o nome "Configuration" e clicar no botão “Add…”.
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace GlobalSist.WMS.ERPExample.Integration { public class Configuration : GlobalSist.WMS.Integration.IntegrationJobInterface { string _ConnectionString { get; set; } //Nullable<DateTime> anchorDate = null; public void Init() { } /// <summary> /// Run the Syncronization Process /// </summary> /// <param name="anchor">Initial Date for the Syncronization Process</param> /// <param name="anchorDate">Date of the Last document syncronized successfully</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool Run(ref string anchor, ref DateTime? anchorDate) { return true; } /// <summary> /// Set Up the Syncronization Process /// </summary> /// <param name="connectionString">Connection String to acces the ERP DataBase</param> /// <param name="company">ERP Company Name</param> /// <param name="username">Username to access the ERP Company</param> /// <param name="password">Password to Access the ERP Company</param> /// <param name="ERP_INSTANCE">ERP Intance</param> /// <param name="ERP_PLATFORMTYPE">ERP Platform Type</param> public void Setup(string connectionString, string company, string username, string password, string ERP_INSTANCE, string ERP_PLATFORMTYPE) { _ConnectionString = connectionString; //Service Registration GlobalSist.ServiceProvider.ServiceManager.AddRegister<DocExtension, Facades.DocLogic.IDoc>(); GlobalSist.ServiceProvider.ServiceManager.AddRegister<BatchExtension, Facades.SKULogic.IBatchFacade>(); GlobalSist.ServiceProvider.ServiceManager.AddRegister<InventoryExtension, Facades.TaskLogic.IInventory>(); GlobalSist.ServiceProvider.ServiceManager.AddRegister<SerialNumberExtension, Facades.SKULogic.ISerialNumberFacade>(); }Deverá, ainda, ser implementado método do DocExtension (podendo também definir os métodos de Batch, Serial e Inventory).
#region IntegrationJobInterface Members public WMS.Integration.IntegrationExtension ERP { //Put here the ERP configured on Eye Peak Params get { return WMS.Integration.IntegrationExtension.PRIMAVERA; } } public Facades.DocLogic.IDoc DocFacade { get { return new DocExtension(); } } public Facades.SKULogic.IBatchFacade BatchFacade { get { return new BatchExtension(); } } public Facades.SKULogic.ISerialNumberFacade SerialNumberFacade { get { return new SerialNumberExtension(); } } public Facades.TaskLogic.IInventory InventoryFacade { get { return new InventoryExtension(); } } #endregion
Passo 4 – Criar classe para extender o tratamento dos documentos
- No nó do projeto clicar, com o botão direito do rato, e escolher Add > New Item…
- Na janela apresentada, expandir na árvore a linguagem pretendida (“C#” ou “VB”).
- Abaixo da linguagem, selecionar Code.
- Selecionar o tipo “Class”.
- Indicar o nome "DocExtension" e clicar no botão “Add…”.
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace GlobalSist.WMS.ERPExample.Integration { internal class DocExtension : Facades.DocLogic.IDoc { #region IDoc Members /// <summary> /// Occurs when the document is closed /// </summary> /// <param name="doc">Doc Object</param> public void OnDocClosed(object doc) { this.OnDocExport(doc); } /// <summary> /// Occurs when the document is canceled or all the lines are removed /// </summary> /// <param name="doc">Doc Object</param> /// <returns>Returns an Object of Type Report. If the object is not empy the operation can be canceled and throw back an error message to the user</returns> public Facades.Base.Report OnDocCanceled(object doc) { //In this example we will return an error message to the user Facades.Base.Report response = new Facades.Base.Report(); Facades.Base.Information info = new Facades.Base.Information(); info.Type = Facades.Base.InformationType.Error; info.Message = "It is not possible to cancel the document"; response.AddInformation(info); return response; } /// <summary> /// Occurs when the document is exported /// </summary> /// <param name="doc">Doc Object</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool OnDocExport(object doc) { // On this example, write a new file on disk string text = "OnDocExport"; System.IO.File.WriteAllText(@"C:OnDocClosing.txt", text); //test //Write your code here return true; } /// <summary> /// Occurs when a new line is added to the document on the Front-End /// </summary> /// <param name="doc">Doc Object</param> /// <param name="lineNumber">Number of the added line</param> /// <returns>Returns an Object of Type Report. If the object is not empy the operation can be canceled and throw back an error message to the user</returns> public Facades.Base.Report OnDocDetailAdding(object doc, int? lineNumber) { //In this example we will return an error message to the user Facades.Base.Report response = new Facades.Base.Report(); Facades.Base.Information info = new Facades.Base.Information(); info.Type = Facades.Base.InformationType.Error; info.Message = "It was not possible to add the new line to the document."; response.AddInformation(info); return response; } /// <summary> /// Occurs when the document is being closed /// </summary> /// <param name="doc">Doc Object</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool OnDocClosing(object doc) { return true; } /// <summary> /// Occurs when the document is being created based on a other document /// </summary> /// <param name="doc">Document to be created</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool OnDocTransformed(object doc) { return true; } /// <summary> /// Occurs when a new line is removed from the document. Only fires for documents which come from the ERP /// </summary> /// <param name="doc">Doc Object</param> /// <param name="line">ID of the line in the ERP (ERPDocDetailID field, set in the documento creation process)</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool OnDocLineDeleted(object doc, string line) { return true; } /// <summary> /// Occurs when a composition is created /// </summary> /// <param name="doc">Doc Object</param> /// <param name="warehouseCode">Warehouse Code</param> /// <param name="skus">List of SKU codes</param> /// <returns></returns> public bool OnCreateComposition(object doc, string warehouseCode, Dictionary<string, decimal> skus) { return true; } /// <summary> /// Indicates if a document is allowed to be ReExported /// </summary> public bool AllowReExport() { return true; } #endregion } }
Passo 4.1 – Criar classe para extender o tratamento dos Lotes
- No nó do projeto carregar com o botão direito do rato, e escolher Add > New Item…
- Na janela apresentada, na árvore expandir a linguagem pretendida (“C#” ou “VB”).
- Abaixo da linguagem, selecionar Code.
- Selecionar o tipo “Class”.
- Indicar o nome "BatchExtension" e carregar no botão “Add…”.
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace GlobalSist.WMS.ERPExample.Integration { internal class BatchExtension : Facades.SKULogic.IBatchFacade { /// <summary> /// Check if a Batch exists in the ERP System /// </summary> /// <param name="skucode">SKU Code</param> /// <param name="batchCode">Batch Code</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool CheckBatchInERP(string skucode, string batchCode) { return true; } /// <summary> /// Check if a SKU Contorls Batch in the ERP System /// </summary> /// <param name="skucode">SKU Code</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool ControlBatchInERP(string skuCode) { return true; } /// <summary> /// Returns a List of Batch available for the SKU in the ERP System /// </summary> /// <param name="skucode">SKU Code</param> /// <returns>Returns a List of Strings</returns> public List<string> GetPrimaveraSkuBatches(string skuCode) { List<string> response = new List<string>(); return response; } } }
Passo 4.2 – Criar classe para extender o tratamento dos Números de Série
- No nó do projeto clicar, com o botão direito do rato, e escolher Add > New Item…
- Na janela apresentada, expandir na árvore a linguagem pretendida (“C#” ou “VB”).
- Abaixo da linguagem, selecionar Code.
- Selecionar o tipo “Class”.
- Indicar o nome "SerialNumberExtension" e clicar no botão “Add…”.
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace GlobalSist.WMS.ERPExample.Integration { internal class SerialNumberExtension : Facades.SKULogic.ISerialNumberFacade { /// <summary> /// Occors when a new Serial Number is created on the documents /// </summary> /// <param name="skuInstance">SKU Object</param> /// <param name="documentInstance">Doc Object</param> /// <param name="serialNumber">Serial Number to be created</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool CheckSerialNumber(object skuInstance, object documentInstance, string serialNumber) { return true; } /// <summary> /// Check if a Serial Number exists on a Document for a specific SKU /// </summary> /// <param name="skuCode">SKU Object</param> /// <param name="documentInstance">Doc Object</param> /// <param name="serialNumber">Serial Number</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool CheckSerialNumber(string skuCode, object documentInstance, string serialNumber) { return true; } /// <summary> /// Check if a Serial Number exists at the ERP System /// </summary> /// <param name="skuCode">SKU Code</param> /// <param name="serialNumber">Serial Number</param> /// <param name="warehouse">Warehoiuse Code</param> /// <returns></returns> public bool CheckSerialNumberInERP(string skuCode, string serialNumber, string warehouse) { return true; } /// <summary> /// Occurs when a Serial Number is Reused. ReUse SerialNumber Option. /// </summary> /// <param name="oldSerialNumber">Old SerialNumber Object</param> /// <param name="reutSerialNumber">New SerialNumber Object</param> /// <returns></returns> public object CreateAbateTask(object oldSerialNumber, object reutSerialNumber) { return reutSerialNumber; } /// <summary> /// Occurs when a Serial Number is Reused. /// </summary> /// <param name="sn">Object containing the SerialNUmber to be Reused.</param> /// <returns>Returns a boolean indicating if the Serial Number can be reused.</returns> public string GetReutSerialNumber(object sn) { GlobalSist.Logic.SKULogic.SerialNumber serialNum = (GlobalSist.Logic.SKULogic.SerialNumber) sn; return serialNum.Serial; } /// <summary> /// Occors when a Serial number is promoted to Principal. Promote to Principal Option /// </summary> /// <param name="reutSN"></param> /// <param name="promotedSN"></param> public void SerialNumberPromoted(object reutSN, object promotedSN) { } } }
Passo 4.3 – Criar classe para extender o tratamento dos Inventários
- No nó do projeto clicar, com o botão direito do rato, e escolher Add > New Item…
- Na janela apresentada, expandir na árvore a linguagem pretendida (“C#” ou “VB”).
- Abaixo da linguagem, selecionar Code.
- Selecionar o tipo “Class”.
- Indicar o nome "InventoryExtension" e carregar no botão “Add…”.
using System; using System.Collections.Generic; using System.Data; using System.Linq; using System.Text; namespace GlobalSist.WMS.ERPExample.Integration { internal class InventoryExtension : Facades.TaskLogic.IInventory { /// <summary> /// Occurs when a new Stock Count is created, and is used to get the Stock of all the SKU's available in the Warehouse /// </summary> /// <param name="warehouseCode">Warehouse Code</param> /// <returns>Should return a Data Table with the following fields: SKU; Batch; Current Stock; Requires Batch; Requires Serial</returns> public DataTable GetERPSkuStocks(string warehouseCode) { throw new NotImplementedException(); } /// <summary> /// Occurs when a SKU is viewed in the Front-End, and returns the SKU Stock from the ERP /// </summary> /// <param name="warehouseCode">Wharehouse Code</param> /// <param name="skuCode">SKU Code</param> /// <param name="batchCode">Batch Code</param> /// <returns></returns> public decimal GetERPStock(string warehouseCode, string skuCode, string batchCode) { throw new NotImplementedException(); } /// <summary> /// Occurs when the Inventory is exported /// </summary> /// <param name="inventory">List of InventoryExport Objects</param> /// <returns>Returns a Boolean which indicates if the operation occured successfully or not</returns> public bool OnInventoryExport(object inventory) { throw new NotImplementedException(); } } }
Passo 5 – Compilar a dll e colocar a mesma na pasta do Serviço
Por último, é necessário compilar a dll para a pasta de instalação (pasta do serviço). Por Exemplo: "C:Program Files (x86)PRIMAVERAWMS510Eye Peak Service". Veja um exemplo sobre a tecnologia de integração com o Eye Peak no GitHub.Esta página foi útil?
Obrigado pelo seu voto.
login para deixar a sua opinião.
Obrigado pelo seu feedback. Iremos analisá-lo para continuarmos a melhorar!
Artigos Relacionados
Como criar um projeto de integração com o Eye Peak via WebServices? Como criar artigos no Eye Peak via WebService? Como criar entidades no Eye Peak via WebService? Como criar armazéns no Eye Peak via WebServices com Visual Studio? Como criar tipos de documentos no Eye Peak via WebServices?